Sagemath
symbolic variables
symbolic functions
interactive plots
animations
show and print
solve
command
Symbolic variables
Symbolic variables are similiar to regular variables. They can be defined using the command var
. They are the building blocks of symbolic expressions.
var(x)
defines a symbolic variable named x. (this is actually done for you by default when you start Sage. Be careful that you do not overwrite this symbolic variable with something else, like a number. You can get unexpected behavior if you are not careful.
DON'T DO THIS!
Symbolic Functions
These are different from regular python functions defined with the def
keyword. Symbolic functions are one-command functions that take variables as arguments. This is probably best illustrated with some examples.
See how f's representation is a function that takes one input and returns one output? Notice how the function's name is just f
, not f(x)
.
We can plot f
by using the plot command.
Interactive Plots
An interactive plot is used when you when to repeatedly try something, visualize your results, and change something again. To create an interactive plot, just put the decorator @interact
over any regularly named function. The interactive plot will repeatedly evaluate your function with the arguments specified by the sliders/checkboxes.
REMEMBER! If you do not use the show
or print
command inside an interactive plot, no output will be displayed.
The syntax for the sliders is similar to srange: (start, end, step_size)
. If you want to have multiple sliders, just give your function multiple arguments. Leaving out the step size argument and just having two arguments makes the slider move continously.
In the example below, the m
slider is continuous and the b
slider goes in increments of 1.
You can also opt to just enter a number into a box. This is convenient if you don't know how fine of a resolution you need.
Animations
An animation is a sequence of figures played back in sequence. It is similar to how a flipbook or a movie works. Use the option gif=True
to have it play back in a nice way for most browsers that won't take up too much bandwidth.
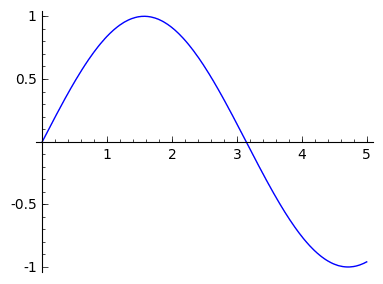
Show and Print
For the most part, show
and print
are almost exactly the same. You can think of show as being a "fancy print". Variables on their own line automatically get print
ed. Also worth noting is that if you want to view output or variables from inside a function or interactive, you'll need to explicitly use the print or show function to view the output.
The solve
Command
The solve
function is used for solving algebraic equations. While it can be used to find symbolic solutions to a single equation, it really shines when used to find solutions to systems of equations. This can be really helpful, for instance, in finding equilibrium points and in determining the number of solutions to a problem when searching for bifurcations. solve
has a somewhat odd syntax and can be a bit clunky to use, but it is very powerful.
As a first example, we'll solve the equation .
Here is the function definition:
"equations" can be either a:
single symbolic expression or a symbolic equation (something with ==)
list of symbolic expressions or symbolic equations.
(We'll call a symbolic equation a symbolic expression that has a ==
in it. ) Symbolic expressions are automatically solved for when they are set equal to zero.
The variables_to_solve_for argument is either a:
single symbolic variable to solve for
list of symbolic variables to solve for These symbolic variables need to have been declared in advance.
We can either look at the output of the solve function very conveniently, but if we want to use the result of the calculation later on in your code, you will need to do a little bit more. As you can see, solve
returns a list. You can access the solutions by indexing the list and calling the rhs()
method on the result.
If this syntax seems odd to you and/or you have multiple variables to deal with, you can use the keyword argument solution_dict=True
to have the solution returned to you as a dict
. Here is an example of solving a system of equations.
We can plot these functions to verify that we have the correct solution. The solution is marked in red.
Plotting points and text
Sagemath has built-in functionality for plotting text and points. Lines and boxes can also be found in the online documentation but are not covered here.